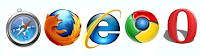
Browser detection
There are many features in web development , which are not supportable with all browsers. Sometimes you need browser detection. Is it a computer browser or a mobile browser? Is it Internet Explorer or Mozilla Firefox? So browser detection is an important issue in the field of website designing. Here are some of the methods I’ve tried and discussed how you can implement them on your websites.
PHP browser detection
You can use php for browser detection and depending on the browser send corresponding html code to clients computer. A few ways discussed below for browser detection using php:
1. Using plugin
Mobile_Detect is a lightweight PHP class for detecting mobile devices. It uses the user-agent string combined with specific HTTP headers to detect the mobile environment. You can visit https://code.google.com/p/php-mobile-detect/ for details.
DOWNLOAD
Latest stable version: https://github.com/serbanghita/Mobile-Detect/tags
WordPress, Drupal, Magento, PrestaShop plugins: https://github.com/serbanghita/Mobile-Detect#projects-that-are-using-the-class
Usage
Include and instantiate the class:
<?phpinclude ‘Mobile_Detect.php’;$detect = new Mobile_Detect();?>
Basic usage, looking for mobile devices or tablets:
<?phpif ($detect->isMobile()) {
// Any mobile device.
}
?>
<?phpif($detect->isTablet()){
// Any tablet device.
}
?>
Check for a specific platform with the help of the magic methods:
<?phpif($detect->isiOS()){
// Code to run for the Apple’s iOS platform.
}
?>
<?phpif($detect->isAndroidOS()){
// Code to run for the Google’s Android platform.
}
?>
Other case insensitive available methods are isIphone(), isIpad(), isBlackBerry(), isKindle(), isOpera(), etc. For the full list of available methods check the demo.php file.
Alternative methods are for checking specific properties (in beta):
<?php$detect->is(‘Chrome’)
$detect->is(‘iOS’)
$detect->is(‘UC Browser’)
[...]
?>
Batch mode using setUserAgent():
<?php$userAgents = array(
‘Mozilla/5.0 (Linux; Android 4.0.4; Desire HD Build/IMM76D) AppleWebKit/535.19 (KHTML, like Gecko) Chrome/18.0.1025.166 Mobile Safari/535.19′,
‘BlackBerry7100i/4.1.0 Profile/MIDP-2.0 Configuration/CLDC-1.1 VendorID/103′,
[...]
);
foreach($userAgents as $userAgent){
$detect->setUserAgent($userAgent);
$isMobile = $detect->isMobile();
$isTablet = $detect->isTablet();
// Use the force however you want.
}
Get the version() of components (in beta):
<?php
$detect->version(‘iPad’); // 4.3 (float)
$detect->version(‘iPhone’) // 3.1 (float)
$detect->version(‘Android’); // 2.1 (float)
$detect->version(‘Opera Mini’); // 5.0 (float)
[...]
?>
You can visit https://github.com/serbanghita/Mobile-Detect#usage for more information.
2. Using $_SERVER['HTTP_USER_AGENT']
You can use the following code to detect computer browsers:
<?phpif(strpos($_SERVER['HTTP_USER_AGENT'], 'MSIE') !== FALSE)
echo 'Internet explorer';
elseif(strpos($_SERVER['HTTP_USER_AGENT'], 'Firefox') !== FALSE)
echo 'Mozilla Firefox';
elseif(strpos($_SERVER['HTTP_USER_AGENT'], 'Chrome') !== FALSE)
echo 'Google Chrome'; elseif(strpos($_SERVER['HTTP_USER_AGENT'], 'Opera Mini') !== FALSE)
echo "Opera Mini"; elseif(strpos($_SERVER['HTTP_USER_AGENT'], 'Opera') !== FALSE)
echo "Opera"; elseif(strpos($_SERVER['HTTP_USER_AGENT'], 'Safari') !== FALSE)
echo "Safari"; else echo 'Something else';
Only for mobile browser detection:
$isMobile = (bool)preg_match('#b(ip(hone|od)|androidb.+bmobile|opera m(ob|in)i|windows (phone|ce)|blackberry'. '|s(ymbian|eries60|amsung)|p(alm|rofile/midp|laystation portable)|nokia|fennec|htc[-_]'. '|up.browser|[1-4][0-9]{2}x[1-4][0-9]{2})b#i', $_SERVER['HTTP_USER_AGENT'] );
if($isMobile) {
//Write some code
}
?>
Browser detection - mobile with tablets:
<?php$isMobile = (bool)preg_match('#b(ip(hone|od|ad)|android|opera m(ob|in)i|windows (phone|ce)|blackberry|tablet'. '|s(ymbian|eries60|amsung)|p(laybook|alm|rofile/midp|laystation portable)|nokia|fennec|htc[-_]'. '|mobile|up.browser|[1-4][0-9]{2}x[1-4][0-9]{2})b#i', $_SERVER['HTTP_USER_AGENT'] );
if($isMobile) {
//Write some code
}
?>
3. Using get_browser()
You can use PHP’s built-in function get_browser(). According to the PHP Manual the output array has platform field which you may use for mobile browser detection.
4. By Mobiforge
There is also a lightweight mobile browser detection code by Mobiforge.
Javascript browser detection
For computer browser detection:
if( /Android|webOS|iPhone|iPad|iPod|BlackBerry/i.test(navigator.userAgent) ){
// some code..
}
Mobile & tablet browser detection:
if (navigator.userAgent.match(/Android/i) ||navigator.userAgent.match(/webOS/i) ||
navigator.userAgent.match(/iPhone/i) ||
navigator.userAgent.match(/iPad/i) ||
navigator.userAgent.match(/iPod/i) ||
navigator.userAgent.match(/BlackBerry/) ||
navigator.userAgent.match(/Windows Phone/i) ||
navigator.userAgent.match(/ZuneWP7/i) ) {
// some code
self.location="top.htm";
}
CSS browser detection
You can use CSS in some browser detection case. Use the following code for mobile browser detection and take some step:
/* Smartphones ----------- */@media only screen and (max-width: 760px) {#some-element {display: none;}}
If you want to serve up CSS just for IE, use a conditional comment.
<link type="text/css" rel="stylesheet" href="styles.css"/><!--for all--><!--[if IE]> <link type="text/css" rel="stylesheet" href="ie_styles.css"/>
<![endif]-->
or if you just want one for IE6:
<!--[if IE 6]> <link type="text/css" rel="stylesheet" href="ie6_styles.css"/> <![endif]-->
Since its a comment its ignored by browsers… except IE that loads it if the if test matches the current browser.
No comments:
Post a Comment